Joomla 4 Templating. Part 5: Templating error.php
In the previous article in the Joomla 4 Templating series, we looked at coding the main template: index.php
In this article, we'll look at templating the error page for our simple
template at: /templates/simple/error.php
. This error page will display a message like 'Oops - that shouldn't have happened!' and 'Find our Homepage here'. I'll also include a graphic: error.jpg
.
Let's trim the fat
Do I really need the entire Bootstrap css/js to style this page? No, what a waste of resources!
Instead I'll use some grid and flex to produce a simple error.css
file which is way smaller than the main site's style.css
. Also, I won't be loading any javascripts. We don't need 'em and it'll make this page load real fast.
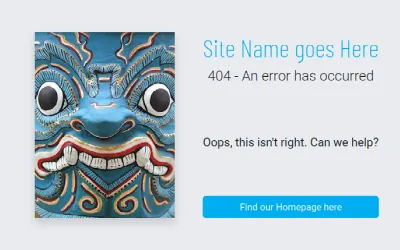
Housekeeping
Before we start, let's add the various assets for this page where they need to go:
- Put
error.jpg
into/media/templates/site/simple/images
- Put
error.css
(anderror.min.css
when you're ready) into/media/templates/site/simple/css
- Add an entry for
error.css
intojoomla.asset.json
joomla.asset.json
Add your error.css
after style.css
, then continue with the rest of the file (not reproduced here).
{
"version": "4.0.0",
"description": "This file contains details of the assets used by simple, a Joomla 4 compatible site template.",
"license": "GPL-2.0-or-later",
"assets": [
{
"name": "template.style.simple",
"description": "The css file to be used for the site.",
"type": "style",
"uri": "style.css"
},
{
"name": "template.error.simple",
"description": "The css file to be used for the error page.",
"type": "style",
"uri": "error.css"
},
<!--continue with rest of entries-->
]
}
The Error File
It's pretty self-explanatory - read the comments in the code.
<?php
defined( '_JEXEC' ) or die;
use Joomla\CMS\Factory;
use Joomla\CMS\HTML\HTMLHelper;
//Use Uri to create home page link
use Joomla\CMS\Uri\Uri;
//Use Text to parse language file strings or insert on the fly text
use Joomla\CMS\Language\Text;
$app = Factory::getApplication();
$wa = $this->getWebAssetManager();
//get sitename
$sitename = htmlspecialchars($app->get('sitename'), ENT_QUOTES, 'UTF-8');
//add the favicon
$this->addHeadLink(HTMLHelper::_('image', 'favicon.png', '', [], true, 1), 'icon', 'rel', ['type' => 'image/png']);
//google fonts - did user paste in google fonts url?
$googlefonts = trim($this->params->get('googlefonts', '', 'string'));
//if so, preload scripts and register/use google fonts
if($googlefonts !=='') {
$this->getPreloadManager()->preconnect('https://fonts.googleapis.com/', ['crossorigin' => 'anonymous']);
$this->getPreloadManager()->preconnect('https://fonts.gstatic.com/', ['crossorigin' => 'anonymous']);
$wa->registerAndUseStyle('googlefonts', $googlefonts, [], ['crossorigin' => 'anonymous'], []);
}
//add a meta tag for the stylesheet
$this->setMetaData('viewport', 'width=device-width, initial-scale=1');
//generate the image tag for the error page
//notice we use Text::_('error') to generate the text for the alt tag, since it's not in the language file
$errorimg = HTMLHelper::_('image', 'error.jpg', Text::_('error'), ['class' => 'img-fluid shadow'], true, 0);
//use the error stylesheet with Web Asset Manager
$wa->useStyle('template.error.simple');
?>
<!DOCTYPE html>
<html lang="<?php echo $this->language; ?>" dir="<?php echo $this->direction; ?>">
<head>
<jdoc:include type="metas" />
<jdoc:include type="styles" />
<jdoc:include type="scripts" />
</head>
<body class="error">
<div class="container">
<div class="box-img">
<!-- make a clickable image to home page -->
<a itemprop="url" title="<?php echo $sitename; ?>" href="<?php echo Uri::base(); ?>">
<?php echo $errorimg; ?>
</a>
</div>
<div class="box-content">
<div class="errtitle">
<h1><?php echo $sitename; ?></h1>
<h3><?php echo $this->error->getCode(); ?> - <?php echo Text::_('An error has occurred'); ?></h3>
<p class="lead"><?php echo Text::_('Oops, this isn\'t right. Can we help?'); ?></p>
</div>
<div class="errbtn">
<a href="<?php echo Uri::base(); ?>" class="btn">
<?php echo Text::_('Find our Homepage here'); ?>
</a>
</div>
</div>
</div>
</body>
</html>
Notes
Although we've inserted some text on the fly like Text::_('An error has occurred')
- it would have been better to have those strings in the template's language file: tpl_simple.ini
. This facilitates changing the text in reusable strings and ofc translations if you're using them.
If you're parsing from the language file, you use it the same way as 'on the fly'. If you had a language string like:TPL_FIND_HOMEPAGE = "Find our Homepage here"
You'd parse it in the template as Text::_('TPL_FIND_HOMEPAGE');
If the string doesn't exist in the language file, the string will print as is, like TPL_FIND_HOMEPAGE
.
What's Next?
In the next article in the Joomla 4 Templating series, we'll finish out the last template in our simple
theme: component.php